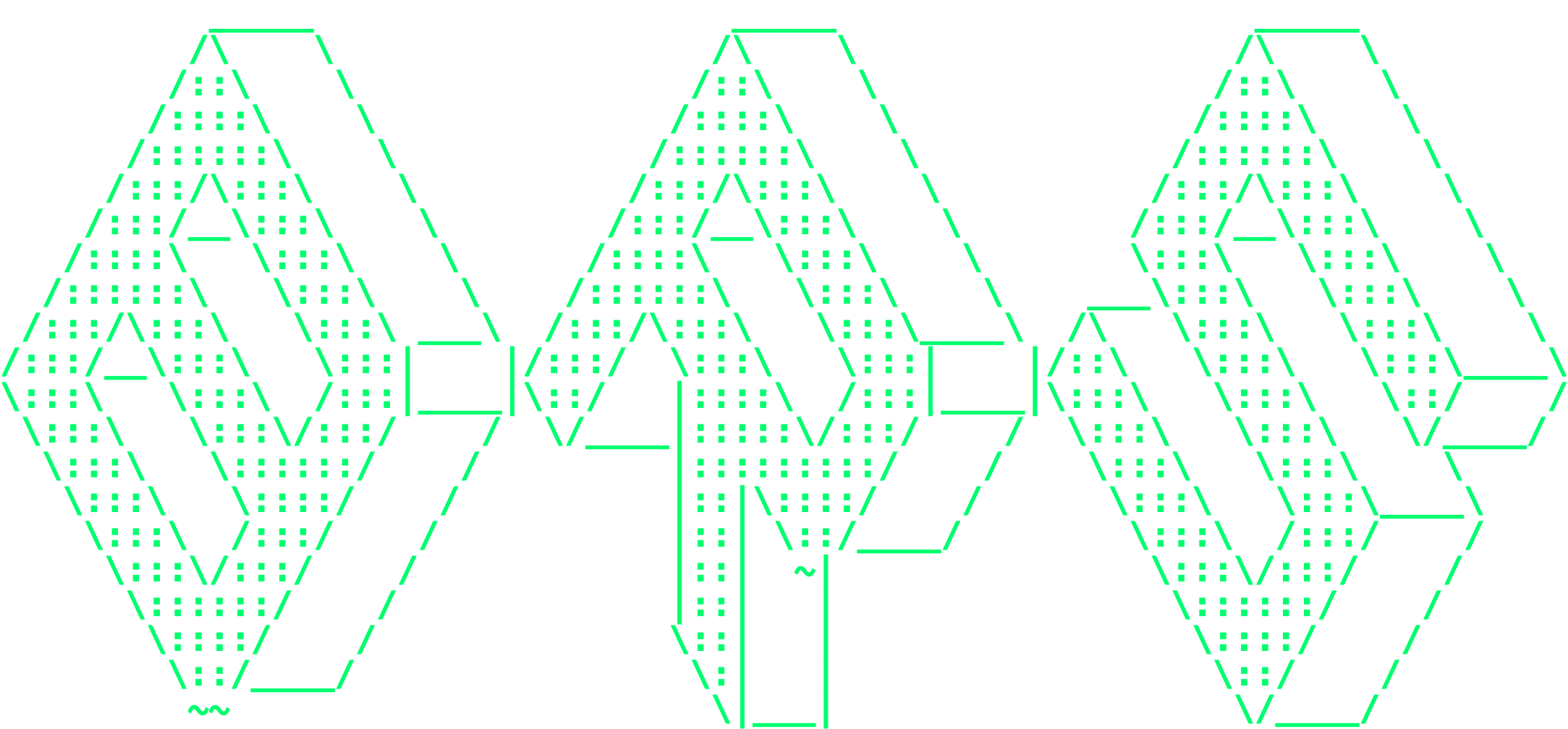
boto3-refresh-session#
Version: 1.0.20
Useful Links: Raison d’Être | Installation | Usage | API Reference | Authorization
Authors: Mike Letts
boto3-refresh-session is a simple Python package for refreshing the temporary security credentials in a boto3.session.Session
object automatically.